Taking Control of the Tab Key with TabIndex & TabEnabled
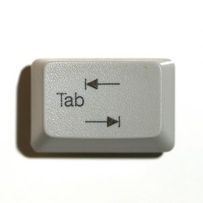
In the world today users will take for granted the ability to use the tab key on the keyboard to navigate between text fields in a form. In Flash you are building dialog boxes from shapes and TextFields so it can't guess how you want the tabs to be controlled. Luckily, you are given total control over this because text fields have the properties tabIndex and tabEnabled.
Tab index – This is an integer that basically allows you to put this text field in a list relative to all the other fields. The first one we should have a tabIndex of one, the second tabIndex of two, and so on. So suppose now you have the text field with tab index of one selected (which for TextFields just means that there is a cursor blinking inside of it). Then pressing tab will move your cursor to the TextField with index two. If you press tab again when the selection is on the text field the highest index then the list will loop back around, and the one that has index one will be selected.
Tab index – This is an integer that basically allows you to put this text field in a list relative to all the other fields. The first one we should have a tabIndex of one, the second tabIndex of two, and so on. So suppose now you have the text field with tab index of one selected (which for TextFields just means that there is a cursor blinking inside of it). Then pressing tab will move your cursor to the TextField with index two. If you press tab again when the selection is on the text field the highest index then the list will loop back around, and the one that has index one will be selected.
var exampleTextField:TextField = new Textfield; exampleTextField.tabIndex = 1;
Tab enabled – This is a boolean property that allows you to toggle the tab feature for textFields. It basically says whether tthe TextField is included in current tabIndex list right now. So suppose you have two pop-ups, and the main pop-up is displayed most of the time. When you bring out the other pop-up, though, that now takes up the whole screen, and you only want your tabbing to cycle through the TextFields on that pop-up that's currently displayed. This is where tabEnabled comes in to save the day. You simply set the tabEnabled properties of all the other TextFields to false, and then set tab enabled property of the text fields on the pop-up currently displayed to true. It is still recommended though that you set tabIndex to 0 when you set tabEnabled to false.
exampleTextField.tabEnabled = true;
Below is an example of a mini state machine that uses the tabEnabled and tabIndex properties to set the tab index for different pop-ups.
public function setTabIndices(loginTabState:String):void { // First turn off tabIndex on all textfields. // Then turn on the ones for the current "loginTabState" disableAllLoginTextFields(); switch (loginTabState) { case "MAIN_POPUP": _loginMc["loginInputTxt"].tabIndex = 1; _loginMc["passInputTxt"].tabIndex = 2; _loginMc["loginInputTxt"].tabEnabled = true; _loginMc["passInputTxt"].tabEnabled = true; break; case "REGISTER_POPUP": _loginMc["registerPopup"]["emailTF"].tabIndex = 1; _loginMc["registerPopup"]["usernameTF"].tabIndex = 2; _loginMc["registerPopup"]["passwordTF"].tabIndex = 3; _loginMc["registerPopup"]["confirmPasswordTF"].tabIndex = 4; _loginMc["registerPopup"]["emailTF"].tabEnabled = true; _loginMc["registerPopup"]["usernameTF"].tabEnabled = true; _loginMc["registerPopup"]["passwordTF"].tabEnabled = true; _loginMc["registerPopup"]["confirmPasswordTF"].tabEnabled = true; break; case "FORGOT_POPUP": _loginMc["forgotPopup"]["forgotInput"].tabIndex = 1; _loginMc["forgotPopup"]["forgotInput"].tabEnabled = true; break; } } private function disableAllLoginTextFields():void { // login pop-up _loginMc["loginInputTxt"].tabIndex = 0; _loginMc["passInputTxt"].tabIndex = 0; _loginMc["loginInputTxt"].tabEnabled = false; _loginMc["passInputTxt"].tabEnabled = false; // forgot pop-up _loginMc["forgotPopup"]["forgotInput"].tabIndex = 0; _loginMc["forgotPopup"]["forgotInput"].tabEnabled = false; // register pop-up _loginMc["registerPopup"]["emailTF"].tabIndex = 0; _loginMc["registerPopup"]["usernameTF"].tabIndex = 0; _loginMc["registerPopup"]["passwordTF"].tabIndex = 0; _loginMc["registerPopup"]["confirmPasswordTF"].tabIndex = 0; _loginMc["registerPopup"]["emailTF"].tabEnabled = false; _loginMc["registerPopup"]["usernameTF"].tabEnabled = false; _loginMc["registerPopup"]["passwordTF"].tabEnabled = false; _loginMc["registerPopup"]["confirmPasswordTF"].tabEnabled = false; }
Here is the official API for Interactive Object.
(TextField extends InteractiveObject and inherits the properties tabIndex and tabEnabled)
(TextField extends InteractiveObject and inherits the properties tabIndex and tabEnabled)
Here are some related articles on tabIndex: